
UI Components
LinkkiTabLayout
Dependency linkki-vaadin-flow-component or linkki-application-framework is needed.
|
The LinkkiTabLayout
is a UI component that gives access to several different views and that allows switching between different contents, using either a horizontal or vertical layout. Only a single tab can be active at a time.
This is a replacement for the linkki Vaadin 8 SidebarSheet
and TabSheetArea
.

Sidebar Layout
To create a LinkkiTabSheet
as sidebar layout, use newSidebarLayout()
, and icons as captions. A description can also be provided which is displayed by hovering the mouse over the icon.
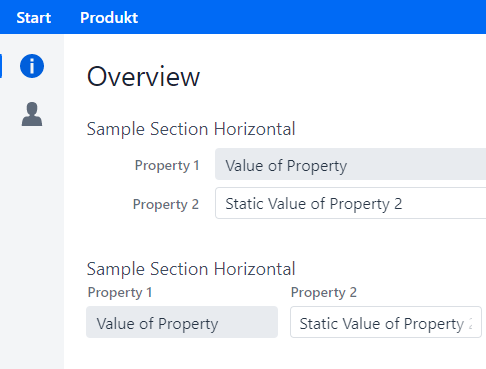
LinkkiTabLayout linkkiTabLayout = LinkkiTabLayout.newSidebarLayout();
linkkiTabLayout.addTabSheet(LinkkiTabSheet.builder("sidebarSheetId")
.caption(VaadinIcon.ABACUS.create())
.description("Sidebar One")
.content(this::createSidebarContent)
.build());
This will result in a vertical bar on the left side containing icons (buttons) for every sheet. The tooltip of the button displays the name of the corresponding sheet. The content of the selected sheet is displayed to the right of the bar.
Creating Tabs
Tabs are added to the layout using a LinkkiTabSheet
. The tab sheet is created using a builder. Every sheet requires an icon, a name and a content supplier (Supplier<Component>
). The supplier is called when the sheet is selected for the first time (lazy initialization). This approach only creates the content if it is requested by the user. It also spreads out the component creation, avoiding long loading times at the start.
LinkkiTabLayout linkkiTabLayout = new LinkkiTabLayout();
linkkiTabLayout.addTabSheet(LinkkiTabSheet.builder("tabSheetId")
.caption("Tab Sheet Caption")
.content(this::createTabContent)
.build());
Toggle Visibility of Tabs
A tab can be dynamically set to visible or invisible by providing a BooleanSupplier
in the builder of LinkkiTabSheet
using the method visibleWhen(BooleanSupplier)
. The suppliers must return true
to set the tab visible and false
to set it invisible.
The method LinkkiTabLayout#updateSheetVisibility()
must be triggered to update the visibility state. The method is automatically called by Vaadin’s AfterNavigationEvent, that means the visibility state is at least updated when there is a navigation to the view containing the tabs.
Selection Change Events
There are several possibilities to receive a selection change event in case a new tab is selected. All events are also triggered for the auto-selection when the first tab is added to the tab layout.
- Implement AfterTabSelectedObserver
-
Components implementing this interface will receive a
TabSheetSelectionChangeEvent
when theLinkkiTabSheet
they are attached to is selected. Its methodafterTabSelected(TabSheetSelectionChangeEvent)
is called every time the sheet is selected, that means it gets visible. Use this observer to update your binding context in case of changes to the underlying model while the sheet was not selected. Additional details and limitations can be found in the javadoc ofAfterTabSelectedObserver
.
AfterTabSelectedObserver
public class ReportListPage extends AbstractPage implements AfterTabSelectedObserver {
@Override
public void afterTabSelected(TabSheetSelectionChangeEvent event) {
getBindingContext().updateUi();
}
- Adding a listener to LinkkiTabLayout
-
Use the method
addSelectedChangeListener(ComponentEventListener<SelectedChangeEvent>)
to get aSelectedChangeEvent
containing the selected tab. - Adding a listener to LinkkiTabSheet
-
It is also possible to add any listener directly to the
LinkkiTabSheet
usingaddTabSelectionChangeListener(ComponentEventListener<TabSheetSelectionChangeEvent>)
even afterwards when adding a PMO as tab component withVaadinUiCreator#createComponent(Object, BindingContext)
toLinkkiTabSheet
. The returnedRegistration
could be used to unregister the listener.